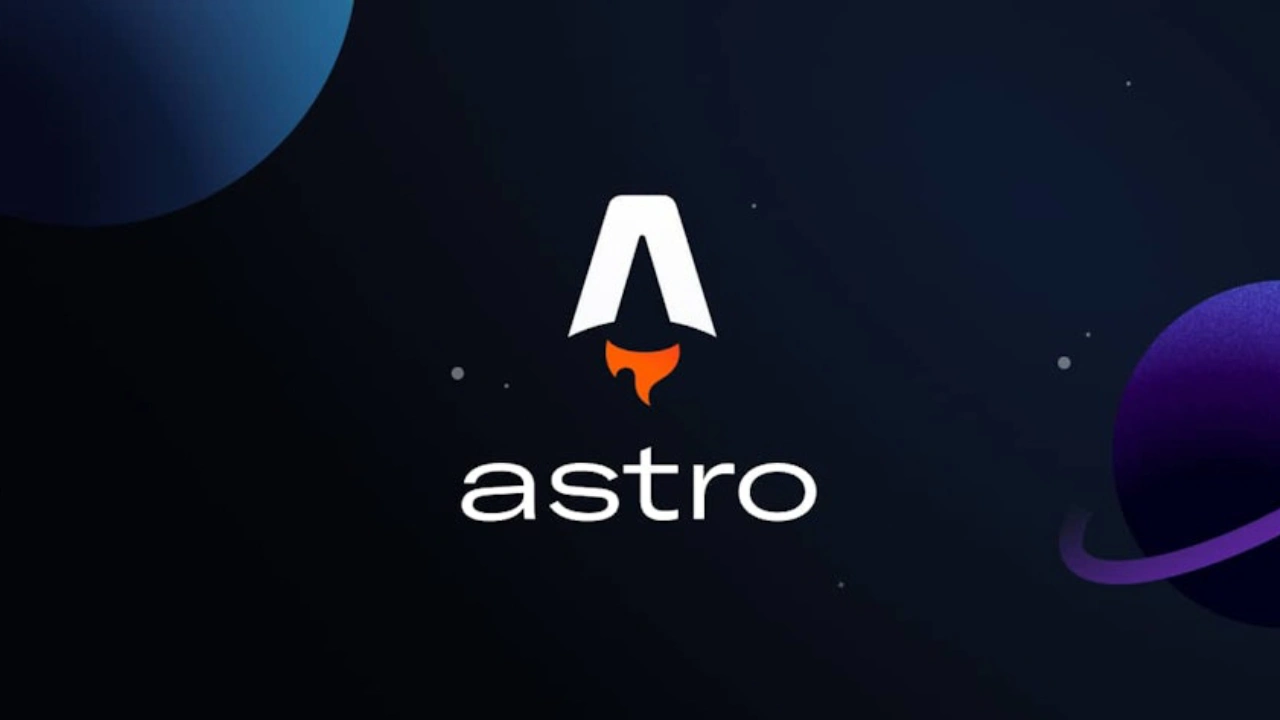
Connecting the WordPress API with Your Astro Website: A Detailed Tutorial
WordPress, with its robust REST API, and Astro, with its focus on static web development, can be combined to create powerful and efficient websites. In this tutorial, we’ll explore how to integrate the WordPress API into an Astro website, allowing retrieval and rendering of dynamic content from WordPress in a static environment.
Step 1: Configuration of the WordPress Theme and API
First, make sure you have a custom theme in WordPress that is configured to expose the necessary data through the WordPress REST API. This involves defining API routes and ensuring that the content you want to retrieve is publicly available.
Step 2: Setting Up the Astro Project
Create a new Astro project or use an existing one. Make sure you have installed the necessary dependencies, such as axios for making HTTP requests.
npm install axios
Step 3: Querying the WordPress API from Astro
In your Astro component files, use axios (or another similar library) to make requests to the WordPress API and retrieve the desired content. For example, to retrieve the latest posts from WordPress, you can do the following:
import axios from 'axios';
const WP_API_URL = 'https://example.com/wp-json/wp/v2';
async function fetchLatestPosts() {
try {
const response = await axios.get(`${WP_API_URL}/posts`);
return response.data;
} catch (error) {
console.error('Error fetching latest posts:', error);
return [];
}
}
Step 4: Rendering Content in Astro
Once you have retrieved data from the WordPress API in Astro, you can render it in your Astro components using JSX. For example, to display a list of the latest WordPress posts, you could do the following:
import React, { useEffect, useState } from 'react';
import { fetchLatestPosts } from './api';
function LatestPosts() {
const [posts, setPosts] = useState([]);
useEffect(() => {
async function fetchData() {
const latestPosts = await fetchLatestPosts();
setPosts(latestPosts);
}
fetchData();
}, []);
return (
<div>
<h2>Latest Posts</h2>
<ul>
{posts.map(post => (
<li key={post.id}>
<a href={post.link}>{post.title.rendered}</a>
</li>
))}
</ul>
</div>
);
}
export default LatestPosts;
Step 5: Practical Example
Suppose you have a portfolio site built with Astro and you want to display the latest posts from your WordPress blog on the homepage. You can include the “LatestPosts” component on your Astro homepage and then deploy your site.
// In the index.astro file
---
import LatestPosts from './components/LatestPosts.astro';
---
This is a practical example of how to integrate the LatestPosts component on the homepage of your Astro website. Now, when you deploy your site, it will automatically display the latest posts from your WordPress blog on the homepage.